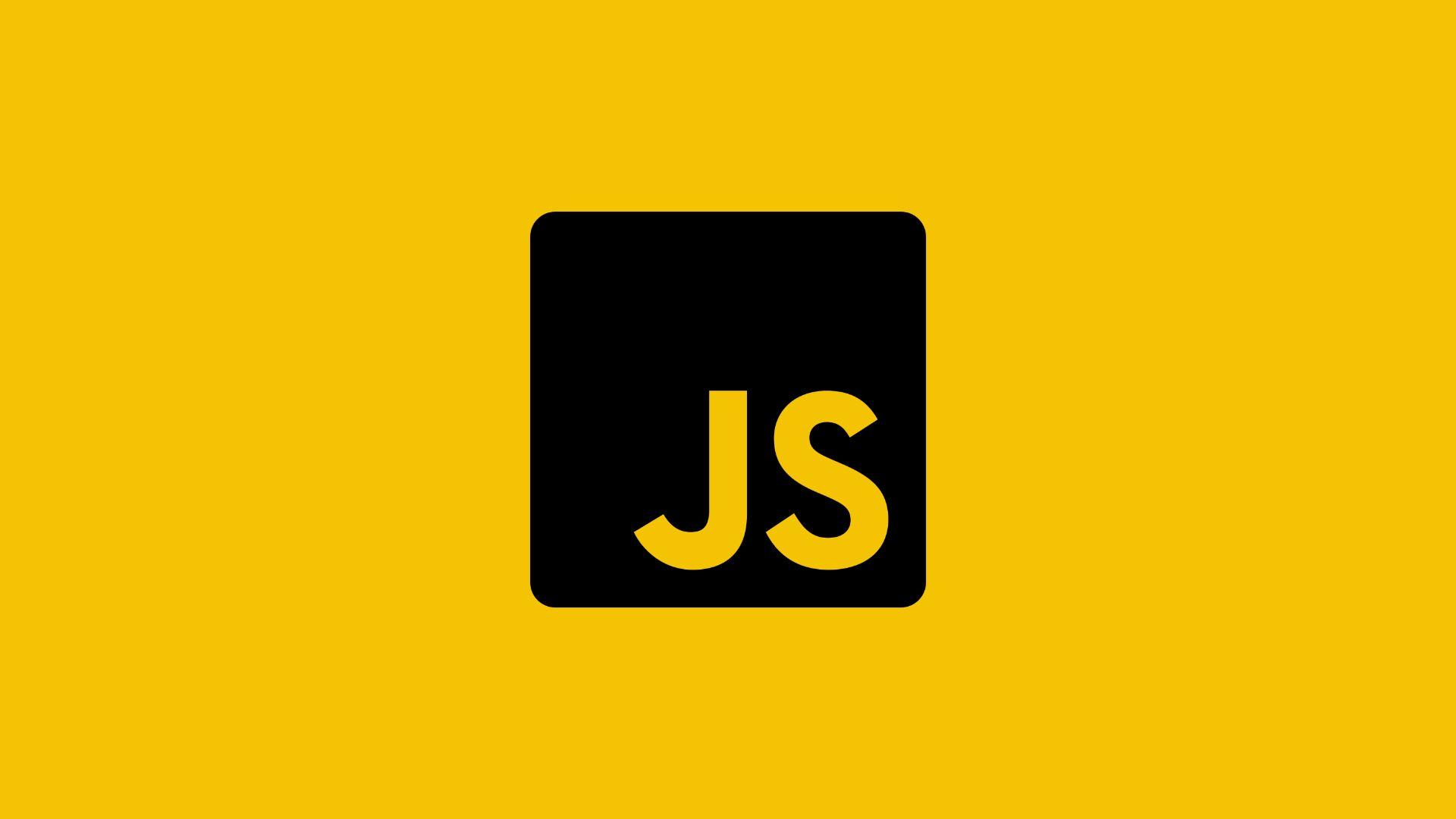
7 JavaScript tips you should know
Published at 17/03/2023 17:00 by Duy Nguyễn.
1 min read
286 views
I will assume I am a salesman. And I will use techniques in JavaScript to improve sales performance.
🔄️ Swap variables
In a conventional way, how would you swap the 2-variable data? Surely you would use a temporary variable to exchange, like the example below, I have a hamburger, and you have chocolate. Can we exchange food?
let myFood = '🍔'
let yourFood = '🍫'
let tempFoot = myFood
myFood = yourFood
yourFood = tempFoot
console.log(myFood, yourFood) // 🍫 🍔
However, you can swap two or more variables with JavaScript without using a temporary variable. And this solution is straightforward.
let myFood = '🍔'
let yourFood = '🍫'
;[ myFood, yourFood ] = [ yourFood, myFood ]
console.log(myFood, yourFood) // 🍫 🍔
See. It's too easy. 😁
☢️ Please stop writing if…else
I need to look up the price of a food by its name. And I will use if...else to be able to compare. But if that pile of food is more than 100 products. I'm going to get a little tired. 😕
Here is the example code:
const getPriceByName = (name) => {
if (name === '🍔') {
return 30
} else if (name === '🍨') {
return 20
} else if (name === '🍿') {
return 10
} else if ...
}
console.log(getPriceByName('🍔')) // 30
Or I can get rid of these meaningless if...else lines. And use an object to be able to save this pile of food and access it faster.
const getPriceByName = (name) => {
const foodMap = {
'🍔': 30,
'🍨': 20,
'🍿': 10,
// You can add new food here
// ...
}
return foodMap[ name ]
}
console.log(getPriceByName('🍔')) // 30
Gosh. I feel better already. 👌
💡 Use Object.entries() wisely
Now if you sell one more product in your store, would you add its name and price, right?
const foodMap = {
'🍔': 30,
'🍨': 20,
'🍿': 10,
'🍫': 5
}
// pay attention here
Object.prototype['🌭'] = 40
Here's how to print out the prices of those products. And unfortunately, I was also printed 🌭. It shouldn't have been there.
// ❌
for (const key in foodMap) {
console.log(key, foodMap[ key ])
}
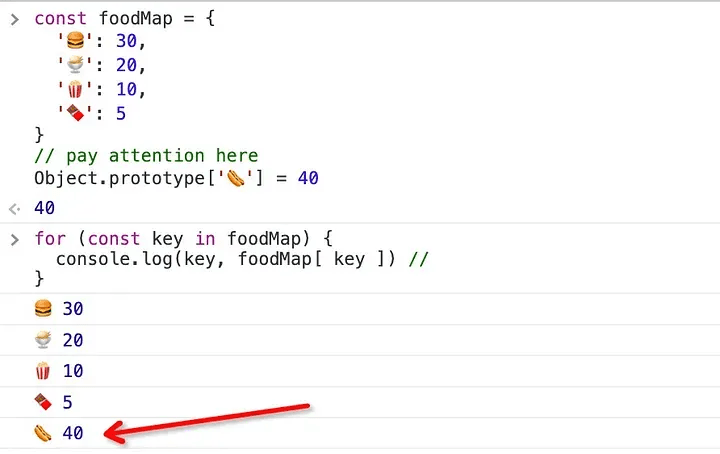
The more efficient way, in this case:
When using Object.entries we can have two strengths which are:
- Properties on `object prototypes` are not printed.
- We can get key and value directly instead of having to use obj[key]
// ✅
Object.entries(foodMap).forEach(([ key, value ]) => {
console.log(key, value)
})
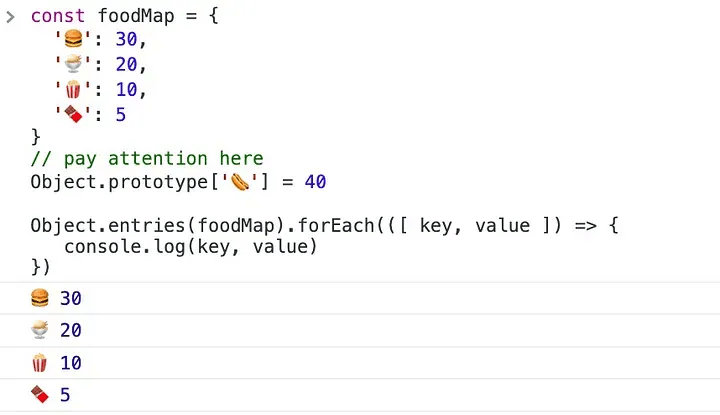
🦀 Flatten an array
It has been a while since I've cleaned up my food. They are arranged in multiple baskets, and it could be more explicit. How can I put them in one basket?
const foods = [ [ '🍔', [ '🍫' ] ], [ '🍨', [ '🍿', [ '🍵' ] ] ] ]
The first way I would use
const flattenFoods = (foods) => {
return foods.reduce((res, food) => {
return res.concat(Array.isArray(food) ? flattenFoods(food) : food)
}, [])
}
console.log(flattenFoods(foods)) // ['🍔', '🍫', '🍨', '🍿', '🍵']
The cool way I often use
The above way feels too complicated and makes me depressed. I think there should be a more straightforward way.
In the mdn web docs, the flat() method creates a new array with all the subarray elements floated into it using recursion until the specified depth.
foods.flat(Infinity) // ['🍔', '🍫', '🍨', '🍿', '🍵']
🧮 Calculate the total price of goods
One of my clients has purchased a large amount of food, and I need to calculate exactly how much he will pay me. That's a lot of money.
First way
const foods = [
{
name: '🍔',
price: 30,
amount: 10,
},
{
name: '🍨',
price: 20,
amount: 3,
},
{
name: '🍿',
price: 10,
amount: 5,
},
{
name: '🍵',
price: 5,
amount: 9,
},
]
let sum = 0
foods.forEach((food) => {
sum += food.price * food.amount
})
console.log(sum) // 455
The way that I will use
Actually, reduce() can quickly get me money. It makes everything look simple, harmonious and only takes one line of code.
const foods = [
{
name: '🍔',
price: 30,
amount: 10,
},
{
name: '🍨',
price: 20,
amount: 3,
},
{
name: '🍿',
price: 10,
amount: 5,
},
{
name: '🍵',
price: 5,
amount: 9,
},
]
let sum = foods.reduce((res, food) => res += food.price * food.amount, 0)
console.log(sum) // 455
📑 Using “console.table()”
I usually use console.log() to print some information, but it's not as clear and concise as the table.
const foods = [
{
name: '🍔',
price: 30.89,
group: 1,
},
{
name: '🍨',
price: 20.71,
group: 1,
},
{
name: '🍿',
price: 10.31,
group: 2,
},
{
name: '🍵',
price: 5.98,
group: 2,
},
]
console.log(foods)
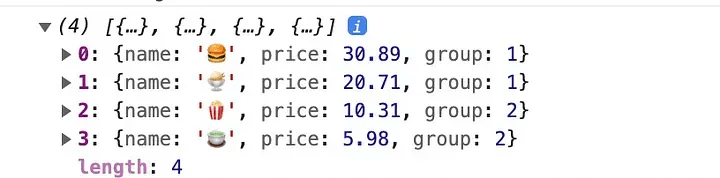
Why don't we try console.table()

Wow, it's intuitive, simple and clear.
↗️ Rounding tricks
To return it to the consumer, our store is promoting all-food discounts and removing the decimals. I must use Math.floor() to calculate the final food price.
So is there a more straightforward way? Actually, yes, it is using the ~~ operator. You can see details here.
const foods = [
{
name: '🍔',
price: 30.89
},
{
name: '🍨',
price: 20.71
},
{
name: '🍿',
price: 10.31
},
]
const discountedFoods = foods.map((it) => {
return {
name: it.name,
price: ~~it.price
}
})
console.log(discountedFoods)
🌟 End
Through the tips that I guide you, I hope you will understand better and be able to apply them in your projects. See yah 🤌.
Tags: codetipjavascriptRealted Posts: